Docker Cheatsheet
I have compiled a small overview of things you need to know for your daily usage of Docker.
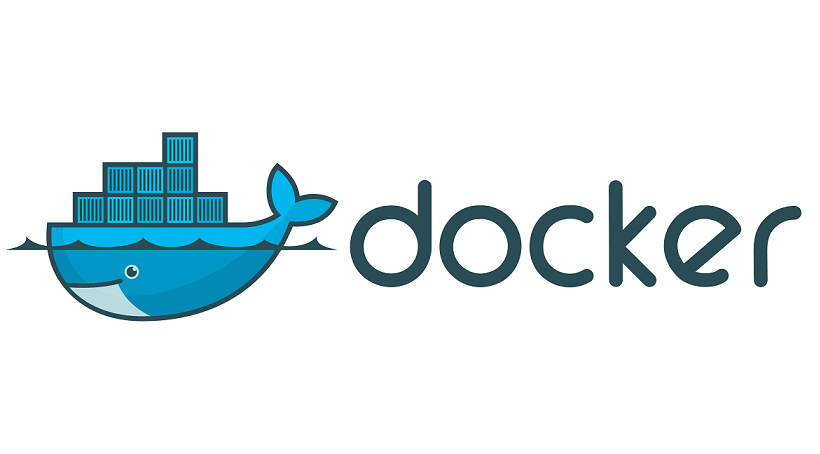
I find the old logo way more appealing
Basic terminology
Docker is a virtualization solution that isolites/separates software inside containers.
There are two words you need to know:
- Image: An application (or multiple ; can be a whole software stack) is translated (build) into an image using a Dockerfile (recipe for the translation).
- Container: An instance of an image. IMPORTANT: All changes made in the container are not persistent by default and will be gone if the container is restarted!
For naming things, you should only use: a - z and 0 - 9 without any special chars except -, _ and .
Administration
General
- Show system information: docker info
- Show live system load: docker stats
Images
- Show all: docker image ls
- Remove specific: docker image rm <id>
Container
- Show all: docker container ls
- Inspect specific: docker container inspect <id>
- Remove specific: docker container rm <id>
Creating / Starting for the first time
- Basic: docker run <image:tag>
- With exposed ports: docker run -p <port_host>:<port_container> <image:tag> (can be multiple ports)
- With a specific network: docker run --network <network_name> <image:tag>
- With a specific name: docker run --name <container_name> <image:tag>
Add -d for a detached start right before the image.
Start/Stop/Restart
- Start: docker start <id>
- Stop: docker stop <id>
- Restart: docker restart <id>
Working inside a container
Getting shell-access into a container:
- On startup: docker run -it <container/image & other options> bash
- After startup: docker exec -it <container-id/name> bash
The -it stands for interactive and bash simply opens a terminal inside the container.
Prune
Deletes unused resources (images, containers, volumes and networks):
- docker image prune
- docker container prune
- docker volume prune
- docker network prune
For deleting everything at once you can use: docker system prune
Mounting a host directory into a container
You have to define that every time you start the container (can't be defined a Dockerfile):
docker run <options> -v Path/on/Host/System:Path/inside/Container <image>
This will mount the host diretory with write access. Hint: If you are on a linux host and only have read-only access inside the container, try the following on the host: sudo chmod -R a+rw YOUR_LOCAL_FOLDER/
If you want the container to only have read only access, append :ro to the end of the path inside the container:
docker run <options> -v Path/on/Host/System:Path/inside/Container:ro <image>
Moving an image from host A to host B
- Save the image on host A into a .tar file: docker save image_name:tag_name > /path/to/save/image.tar
- Copy the .tar to host B.
- Import the image using: docker load < image.tar
Running a local registry
You can run your own, private registry for storing, pulling and distributing images in your own network.
The registry is a simple container and only needs to be pulled from the Docker-Hub:
docker run -d -p 5000:5000 --restart always --name registry registry:2
The -d runs the container in detached mode (meaning it will run it the background), the -p will expose the container port 5000 on the host on port 5000 and the rest should speak for itself.
Viewing the catalog of images
This example uses curl: curl -X GET http://localhost:5000/v2/_catalog
Pushing images
- docker tag your_docker_image your_hostname:5000/your_docker_image
- docker push your_hostname:5000/your_docker_image
Pulling images
Use this command: docker pull your_hostname:5000/your_docker_image
HTTPS
If you use HTTP for pulling, you will get this warning: http: server gave HTTP response to HTTPS client
To bypass that, go to /etc/docker/daemon.json and append the following to the JSON object (at root level): "insecure-registries": ["your_hostname:5000"]
After that, restart the docker daemon.
Networking
Usually there are multiple networks (bridged):
- 172.17.0.0/16 Standard, the host has 172.17.0.1
- 172.18.0.0/16, the host has 172.18.0.1
Networks in general
- Show all: docker network ls
- Inspect specific: docker network inspect <id>
- Remove specific: docker network rm <id>
Create a new network
docker network create \
--driver=bridge \ #Defines a bridge for a physical network adapter
--opt com.docker.network.bridge.name=docker1 \ #Name of the network (visible in the os)
--subnet=10.10.0.0/24 \ #Address space for the new network (internal)
--gateway=10.10.0.1 \ #The Gateway for every container on the network (internal)
--opt "com.docker.network.bridge.host_binding_ipv4=10.8.0.1" \ #The IP address of the interface, the bridge is supposed to use
network_name #Name of the network (visible in docker)
You can also define additional DNS entries for a container. Use the --add-host domain.tld:bind-ip-Parameter with docker run.
For firewalling, you have to take a look at iptables, because the well known and easy to use ufw sadly doesn't work in combination with Docker.
The list is definitely not complete, but I might add more stuff to it later.